1
/
of
1
REJEKIPOKER
REJEKIPOKER | Daftar Situs Judi Bandarq Online Pkv Games Terpercaya
REJEKIPOKER | Daftar Situs Judi Bandarq Online Pkv Games Terpercaya
Regular price
Rp 0,00 IDR
Regular price
Sale price
Rp 0,00 IDR
Unit price
/
per
Couldn't load pickup availability
Bandarq adalah situs judi online yang menyediakan permainan judi deposit uang asli gampang dimenangkan bersama situs pkv games terpercaya. Dengan deposit termurah sudah bisa bermain permainan bandarq di situs pkv games yang menyediakan banyaknya permainan gampang menang dan pastinya terpercaya di Indonesia. Segera lakukan pendaftaran dan dapatkan permainan gampang menang bersama situs dominoqq pkv games terpercaya dan gampang menang di Indonesia.
Share
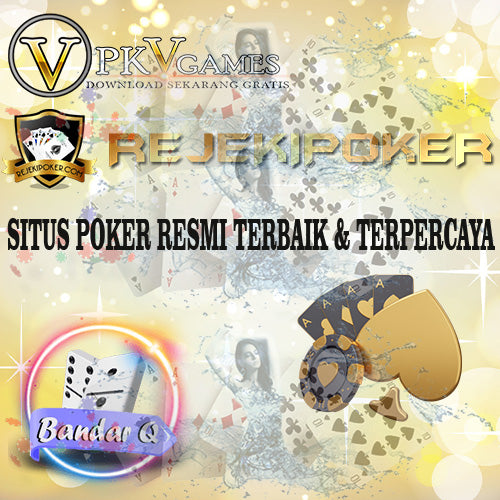